A hacked-together Python command-line Audio Player
The other day it occurred to me to see if there were any good terminal-based audio players since the last time I looked.
There weren't, at least not in the goldilocks zone that I have in mind, which is a little more than mplayer
and a lot less than cmus
. All I want is a terminal command that takes some audio filenames and plays them with a nice TUI and basic start/pause/stop/skip controls.
While poking around today I found the Python audioplayer library, and it didn't take much to get a script working that will:
- Load audio files from a directory
- Play them in order, using tqdm to show a progress bar as they go.
from audioplayer import AudioPlayer
import os
from time import time
from tqdm import tqdm
ALBUM = './Optiganally Yours/O.Y. In Hi-Fi'
for f in sorted(os.listdir(ALBUM)):
if f[-4:] == 'flac':
p = AudioPlayer(f"{ALBUM}/{f}")
# (There might be a way to make the player load the file without
# the play/pause step, but I haven't dug too deeply)
p.play()
p.pause()
dur = round(p._player.duration())
started_at = time()
tick = started_at
p.play()
pbar.update(1)
is_playing = True
with tqdm(total=dur, desc=f.rjust(25, ' ')) as pbar:
while round(p._player.currentTime()) <= dur and is_playing:
now = time()
if now - tick >= 1:
pbar.update(1)
tick = now
if tick - started_at >= dur:
is_playing = False
p.stop()
p.close()
pbar.close()
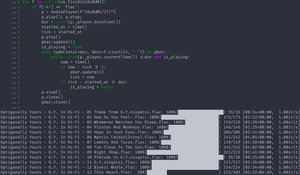
I also discovered blessed, which could be used to build an actual interface.
Prerequisites
You'll need gstreamer installed on your system, and the following Python packages:
audioplayer
tqdm
I ran this right from a REPL, but it ought to work as a script.
Tue Jul 09 2024 20:00:00 GMT-0400 (Eastern Daylight Time)